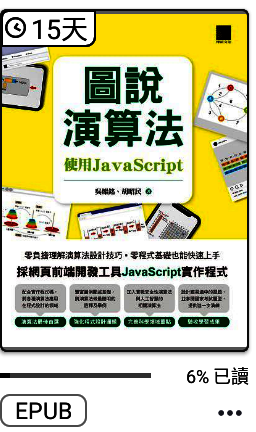
3-7題目:將句中或片語單字反轉 Reverse Words
給定一個短句,將句子中的每一個單字的字母順序反轉,請注意,是句子中的每個單字,以字做為單位進行字母順序反轉。
JS reverse_word.js
const change = str =>{
const answer = [];
str.split(" ").forEach(word =>{ //注意""有空格
let temp = ""; //注意""沒空格
for(let i = word.length-1; i>=0; i--){
temp +=word[i];
}
answer.push(temp);
});
return answer.join(" "); //注意""有空格
};
console.log("原來句子:");
console.log("The greatest test of courage on earth is to bear defeat without losing heart.");
console.log("句子中每個單字都反轉:");
console.log(change("The greatest test of courage on earth is to bear defeat without losing heart."));
PHP reverse_word.php
$my_str="The greatest test of courage on earth is to bear defeat without losing heart.";
function my_reverse ($str){
$my_arr = explode(" ", $str);
$len = count($my_arr);
$i = 0;
while ($i<=$len-1){
$t_str[]=strrev($my_arr[$i]);
$i++;
}
$my_re=join(" ",$t_str);
return $my_re;
}
$my_re = my_reverse($my_str);
echo $my_str."<br>";
echo $my_re;
使用函數:
explode()
count()
join() 或 implode() 把陣列元素组合成的字符串。
strre() 反轉字串
3-8題目:首字大寫 Capitalization
給定一個短句,將句子中的每一個單字的第一個字母轉為大寫,請注意,是每句子中的每一個單字,以字做為單位進行首字大寫轉換。
JS capitalization.htm
const bigletter = sentence => {
const words = []; //宣告words陣列
for (let word of sentence.split(""))
words.push(word[0].toUpperCase() + word.slice(1));
return words.join("");
};
console.log("原來的句子");
console.log("Genius is an infinite capacity for taking pains.");
console.log("首字大寫的句子:");
console.log(bigletter("Genius is an infinite capacity for taking pains."));
PHP capitalization.php
$my_str="Genius is an infinite capacity for taking pains.";
function my_bigletter ($str){
$my_arr = explode(" ",$str);
$my_len = count($my_arr);
$t_big = array();
$i = 0;
while ($i<=$my_len-1){
array_push($t_big, ucfirst($my_arr[$i]));
$i++;
}
$f_big = implode(" ", $t_big);
return $f_big;
}
$f_bigletter=my_bigletter($my_str);
echo $f_bigletter;
使用函數
explode()
count()
array_push() 将一个或多个元素插入陣列的尾端
implode()
3-9平均值 Mean
給定一個數字組,計算平均值,例如給定的陣列[1,2,3],則回傳平均值為2.0。這支程式可以利用Javascript的reduce()方法,它能將陣列中每項元素(由左至右)傳入回呼函式,將陣列化為單一值。
JS mean.js
const stat1 = [2,8,7,6,5,6,4];
const stat2 = [9,2,8,7,6,5,6,4];
const reducer = (accu, currentValue) => accu + currentValue;
//2,8,7,6,5,6,4
console.log("第1組原始資料");
console.log("[2,8,7,6,5,6,4]");
console.log(stat1.reduce(reducer)/stat1.length);
//9,2,8,7,6,5,6,4
console.log("第2組原始資料");
console.log("[9,2,8,7,6,5,6,4]");
console.log(stat2.reduce(reducer)/stat2.length);
PHP mean.php
$my_arr_1=array(2,8,7,6,5,6,4);
$my_arr_2=array(9,2,8,7,6,5,6,4);
echo "數字陣列1:";
print_r($my_arr_1);
echo "<br>";
echo "數字陣列2:";
print_r($my_arr_2);
echo "<br>";
function get_mean ($my_arr){$len = count($my_arr);$total = array_sum($my_arr);
$mean = $total/$len;return $mean;
}
$my_mean_1 = get_mean($my_arr_1);
$my_mean_2 = get_mean($my_arr_2);
echo "my_mean_1=".$my_mean_1."<br>";
echo "my_mean_2=".$my_mean_2."<br>";
使用函數
count()
array_sum() 計算陣列元素值的總和
3-10回傳給定總和的數值序對 Two Sum
這個函數會傳入兩個引數,第一個引數是一個包含多個數字的整數值,第二個引數為給定的總和值(sum),該函數會回傳所有加總起來會等於給定總和的所有數值序對,這個數值序對內的數字可以多次使用。例如給定的第一個引數為[1,2,2,3,4],給定的第二個引數為數值4,則陣列中的3及1加總結果為4。另一種狀況,陣列中的2及2加總結果為4,則回傳的結果值為[[2,2],[3,1]]。
JS two_sum.js
const twototal = (num, total) =>{
const subpair = [];
const ans = [];
for (let a of num) {
const b = total -a;
if (ans.indexOf(b) !== -1){
subpair.push([a,b]);
}
ans.push(a);
}
return subpair;
}
console.log ("第1組原始資料");
console.log ("twototal([1,2,2,3,4], 4)=");
console.log (twototal([1,2,2,3,4], 4));
console.log ("第2組原始資料");
console.log ("twototal([2,3,5,1,5,1,3,4], 6)=");
console.log (twototal([2,3,5,1,5,3,4], 6));
Array.prototype.indexOf() 找出元素索引值的陣列indexOf()
Array.prototype.push() 會將一或多個的值,加入至一個陣列中
PHP two_sum.php
$arr_1 =array (2,3,5,1,5,1,3,4);
function get_two_sum($arr, $num){
$t_arr = array();
$ans = array();
$len = count($arr);
$i=0;
while ($i <=$len-1){
$b = $num - $arr[$i];
$arr_slice = array_slice($arr, $i+1);
if (in_array($b, $arr_slice) == true){
$ans[]=array($arr[$i], $b);
}
$i++;
}
return $ans;
}
$my_two_sum = get_two_sum($arr_1, 6);
print_r($my_two_sum);
使用函數
count()
arr_slice($arr,$num) 函數切割 $arr陣列、$num從多少開始切
in_array($val,$arr) 在函數中尋找是否有值