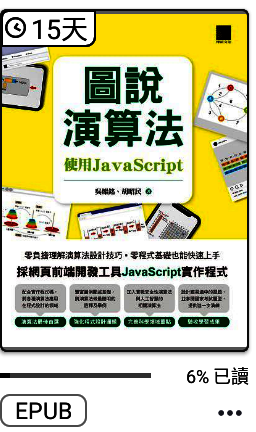
3-11最大利潤 Max Profit
給定一系列股票價格,找出產生最大利潤的最小買入價和最大賣出價。舉例以陣列的方式提供一系列的股票價格,例如[24,27,32,36,45],這個函數會找出這一系列的股票價格的最低買入價及最高賣出價,再以陣列的方式回傳,如此一來可以計算出這支股票的最大利潤。
JS max_prifit.js
var maxProfit=(prices)=> {
let low = prices[0] < prices[1]? prices[0]:prices[1];
high = prices[0] < prices[1] ? prices[1]:prices[2];
let maxProfit = high - low;
let temp = low;
for (let index = 2; index < prices.length; index++){
const sellPrice = prices[index];
if (low > sellPrice) temp = prices[index];
else{
const profit = sellPrice - low;
if (profit > maxProfit)
(maxProfit = profit),
(high = sellPrice),
(low = temp);
}
}
return [low, high];
}
console.log("產生最大歷潤的最小買入價和最大賣出價分別為:");
console.log(maxProfit([24,27,32,36,45]));
PHP max_profit.php
$arr= array (24,27,32,36,45);
function get_max_profit($arr){
$low = ($arr[0] < $arr[1]) ? $arr[0] : $arr [1];
$high = ($arr[0] > $arr[1]) ? $arr[0] : $arr[1];
$maxProfit = $high - $low;
$temp = $low;
$len = count($arr);
$ans = array();
$i=1;
while ( $i <= $len-1){
$sellPrice = $arr[$i];
if($low > $sellPrice) $temp = $arr[$i];
else {
$profit = $sellPrice -$low;
if($profit > $maxProfit){
$maxProfit = $profit;
$high = $sellPrice;
$low = $temp;
}
}
$i++;
}
$ans[] =array($low,$high);
return $ans;
}
$my_max_profix = get_max_profit($arr);
print_r($my_max_profix);
3-12費伯納序列 Fibonacci
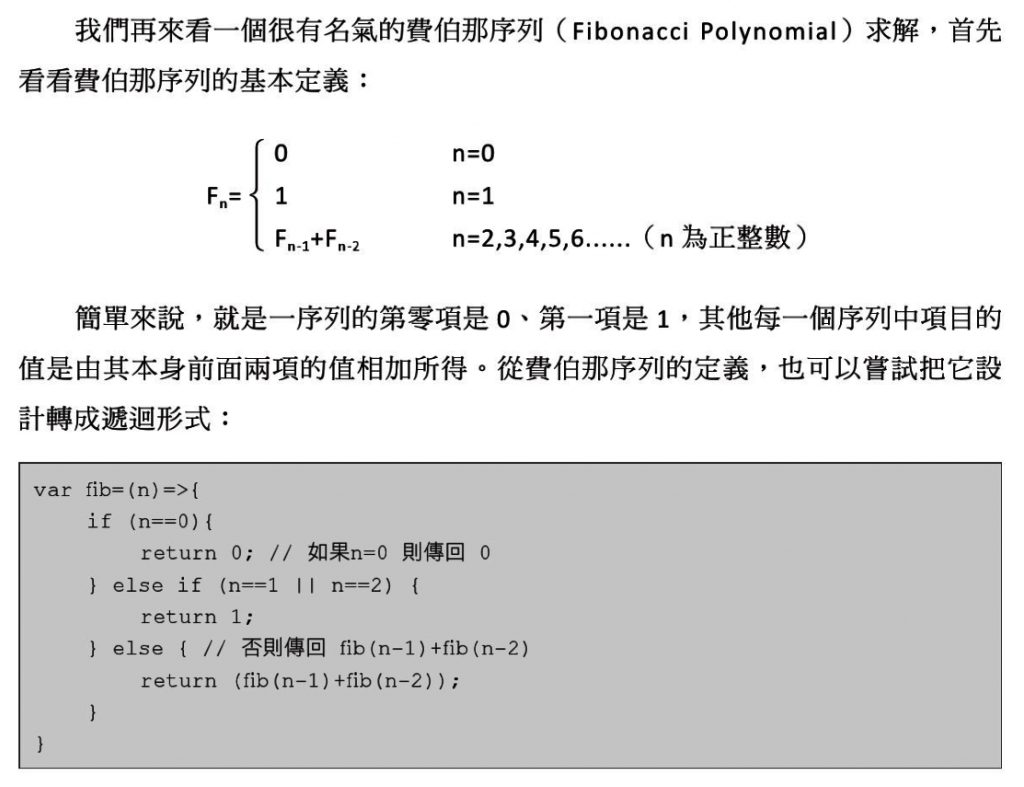
JS fibonacci.js
var fib=(n)=>{
if (n==0){
return 0;
} else if (n==1 || n==2){
return 1;
} else{
return (fib(n-1)+fib(n-2));
}
}
const num=10;
for(i=0; i<=num; i++){
console.log("fib("+i+")=" + fib(i));
}
PHP fibonacci.php
$my_num = 30; //超過38時,執行會超過30秒
function my_fibnacci ($n){
switch ($n) {
case ($n==0):
return 0;
break;
case ($n==1 ||$n==2):
return 1;
break;
default:
return(my_fibnacci($n-1)+my_fibnacci($n-2));
break;
}
}
for ($i=0 ; $i<=$my_num; $i++){
echo "fib(".$i.")=".my_fibnacci($i)."<br>";
}
3-13記憶式費伯納序列 Memoized Fibonacci
這是一種具有記憶性質的費伯納序列的做法,它可以使得在求取費伯納序列能以較高的效率取得。也就是動態規劃法,它是一種具備記憶體功能的演算法。
JS momoized_fibonacci.js
output =[]; //宣告陣列
var fib=(n)=>{
if (n==0) return 0;
if (n==1 ) return 1;
else{
output[0]=0;
output[1]=1;
for (t=2; t<=n; t++){
output[t]=output[t-1]+output[t-2];
}
return output[n];
}
}
const num=9;
for(i=0; i<=num; i++){
console.log("fib("+i+")=" + fib(i));
}
PHP memoize_fibonacci.php
$my_num = 50;
function my_fibnacci ($n){
$ans=array();
if($n==0) return 0;
if($n==1) return 1;
else{
$ans[0]=0;
$ans[1]=1;
for ($j=2; $j<=$n; $j++){
$ans[$j]=$ans[$j-1]+$ans[$j-2];
}
return $ans[$n];
}
}
for ($i=0 ; $i<=$my_num; $i++){
echo "fib(".$i.")=".my_fibnacci($i)."<br>";
}